Internationalization operations
Check out this article and learn more details about the WEBfactory 2010 Client SDK internationalization operations and the involved paramters.
The following article documents the Internationalization methods from the WEBfactory 2010 Silverlight SDK. To view an example on how to use the Internationalization methods, click on the link below:
RegisterLanguageChangedHandler
Method Definition
void RegisterLanguageChangedHandler(string serverName, string[] symbolicNames, OnLanguageChangedDelegate onLanguageChangedDelegate);
Calling Parameters
serverName Host name of WEBfactory 2010 server
symbolicNames Array of symbolic names
onLanguageChangedDelegate Handler for language changes
Description
This method assigns a delegate that is executed if the language changed.
serverName is necessary for multi server systems only. For a single server project it can be empty.
The delegate onLanguageChangedDelegate is defines as:
void OnLanguageChangedDelegate(string[] symbolicNames, string[] translationTexts);
translationTexts is a string array that has a matching index to symbolicNames array. That means for each symbolic text there is one translation in the current language.
ReadLanguages
Method Definition
WFLanguage[] ReadLanguages(string serverName);
Calling Parameters
serverName Host name of WEBfactory 2010 server
Description
This method returns all available languages from database.
serverName is necessary for multi server systems only. For a single server project it can be empty.
The returned array can be null if the system did not receive all languages yet. ReadLanguages can be used after the first language changed delegate is invoked.
Return parameter is an array of type WFLanguage:
public class WFLanguage { public int LanguageId; public string Description; public bool IsActive; public bool IsDefault; }
LanguageId is unique identifier as an integer value for the language.
(German = 7, English = 9 …).
Description is the plain text of the name of the language.
IsActive is a Boolean value that defines whether this language is currently active or not.
IsDefault is true if the language is the WEBfactory 2010 default language.
GetLanguageId
Method Definition
int GetLanguageId();
Calling Parameters
None
Description
This method returns the language identifier of the current language.
The returned integer can be -1if the system did not initialize yet. GetLanguageId can be used after the first language changed delegate is invoked.
GetCurrentCulture
Method Definition
CultureInfo GetCurrentCulture();
Calling Parameters
None
Description
This method returns the CultureInfo of the current language.
The returned CultureInfo is part of the System.Globalization namespace and it is used for displaying formatted data.
SetLanguageId
Method Definition
void SetLanguageId(int languageId);
Calling Parameters
languageId language identifier
Description
This method sets the language identifier of the current language.
SetTranslationServer
Method Definition
void SetTranslationServer(string serverName);
Calling Parameters
serverName Host name of WEBfactory 2010 server
Description
This method sets the WEBfactory 2010 2006 translation server in a multi-server environment.
For a single WEBfactory 2010 server project it is not necessary to invoke this method.
Internationalization operations tutorials
Check out these easy step based guides in order to learn how te WEBfactory 2010 Client SDK internationalization operations work.
In the following tutorial, you will learn to extend the ClientSDKExample Silverlight Application to display a symbolic text in different languages and change the language at run time. For this, we will add three new controls to the project: a text block and two buttons.
The following tutorial is based on he solution build in the Write operations Example tutorial.
In order to complete the steps described below, WEBfactory 2010 Server must be running and the Demo database must be loaded in WEBfactory 2010Studio.
All versions of Microsoft Visual Studio and Microsoft Expression Blend can be used to create this example. For extended compatibility, we will use Microsoft Visual Studio 2010.
Also the symbolic text SymbolicText must be defined in the WEBfactory 2010Studio project and translated in German and English.
The guide is split in three sections:
The final solution is available for download here:
Make sure to unblock any downloaded file before using it. Windows automatically blocks the files coming from the internet for security reasons.
If downloading a Visual Studio solution, make sure to update all the references marked with the warning symbol before building it.
Editing the XAML
To demonstrate the Internationalization operations available in the Client SDK, we need to use another three controls: on TextBlock to hold the symbolic text that will be translated and two buttons to allow us to switch between the two languages we will use. The same operations need to be performed in both Visual Studio and Expression Blend.
Using Microsoft Visual Studio
In the ClientSDKExample solution, go to the Solution Explorer and open the MainPage.xaml.
In the horizontal StackPanel container, add a new vertical StackPanel to hold our new controls. Set the Margin attribute to 2.
<StackPanel Orientation="Vertical" Margin="2"> </StackPanel>
In this new StackPanel, add a new TextBlock control and set its Text attribute to Internationalization and its FontWeight attribute to Bold:
<StackPanel Orientation="Vertical" Margin="2"> <TextBlock Text="Internationalization" FontWeight="Bold"></TextBlock> </StackPanel>
Add a new TextBlock and set its Name property to SymbolicTextLabel.
<StackPanel Orientation="Vertical" Margin="2"> <TextBlock Text="Internationalization" FontWeight="Bold"></TextBlock> <TextBlock Name="SymbolicTextLabel"></TextBlock> </StackPanel>
Now add two Button controls. The first one must have the Name attribute set to ENButton and the Content attribute set to EN. The second one must have the Name attribute set to DEButton and the Content attribute set to DE.
<StackPanel Orientation="Vertical" Margin="2"> <TextBlock Text="Internationalization" FontWeight="Bold"></TextBlock> <TextBlock Name="SymbolicTextLabel"></TextBlock> <Button Name="ENButton" Content="EN"></Button> <Button Name="DEButton" Content="DE"></Button> </StackPanel>
We also need to define the OnClick events for each of the two buttons:
<Button Name="ENButton" Content="EN" Click="ENButton_Click"></Button> <Button Name="DEButton" Content="DE" Click="DEButton_Click"></Button>
Using Microsoft Expression Blend
Using the Assets panel, add the following three controls on the MainPage: two TextBlock controls and two Button controls.
Set the text of the first TextBlock to Internationalization and make its font Bold.
Set the name of the second TextBlock control to SymbolicTextLabel using the Name property from the Properties panel.
Set the texts for the two buttons to EN and DE and name them ENButton and DEButton using the Name property.
For both ENButton and DEButton, use the Events tab of the Properties panel to create a Click event. Double-click in the Click event to automatically add the event handlers.
Programming the application
The programming part of the ClientSDKExample goes the same for both Microsoft Visual Studio and Expression Blend, though it is highly recommended to use Microsoft Visual Studio for programming.
Use the Solution Explorer to open the MainPage.xaml.cs.
Define a new string array that will hold the symbolic texts (under the SignalName constant). The RegisterLanguageChangedHandler method requires an array of strings to get the symbolic texts. This way, the method is not limited to getting one single symbolic text, but any number of them. We also need to populate the array with our symbolic text:
private string[] MySymbolicTexts = new string[] { "SymbolicText" };
On the UserControl_Loaded event, we need to register the connector to the RegisterLanguageChangedHandler event that will execute the
OnLanguageChanged()
delegate method. This action needs to happen at run time only, so the code will go under the if statement, next to RegisterSignalChangedHandler:wfConnector.RegisterLanguageChangedHandler(MySymbolicTexts, OnLanguageChanged);
Now we need to create the
OnLanguageChanged()
method that will return an array of translation texts and write it in the Text property of the SymbolicTextLabel TextBlock:private void OnLanguageChanged(string[] symbolicNames, string[] translationTexts) { SymbolicTextLabel.Text = translationTexts[0]; }
In the DisposeConnector method, we need to unregister the connector from the LanguageChanged event when the application is closed, to avoid memory leaks:
wfConnector.UnregisterLanguageChangedHandler(MySymbolicTexts, OnLanguageChanged);
Programming the Buttons to switch the language at run time
To toggle the languages at run time, we need to use the SetLanguageId method from the WEBfactory 2010 Silverlight SDK to set the language IDs corresponding to our two buttons (9 is English, 7 is German). We will use the Click events of our buttons to call the SetLanguageId()
method (next to the OnLanguageChanged event):
private void ENButton_Click(object sender, RoutedEventArgs e) { wfConnector.SetLanguageId(9); }
We will do the same for the De button:
private void DEButton_Click(object sender, RoutedEventArgs e) { wfConnector.SetLanguageId(7); }
Building and testing the application
Press F5 to build and run the solution. Make sure that the correct database (containing the used signals) is loaded and the WEBfactory 2010 Server is started.
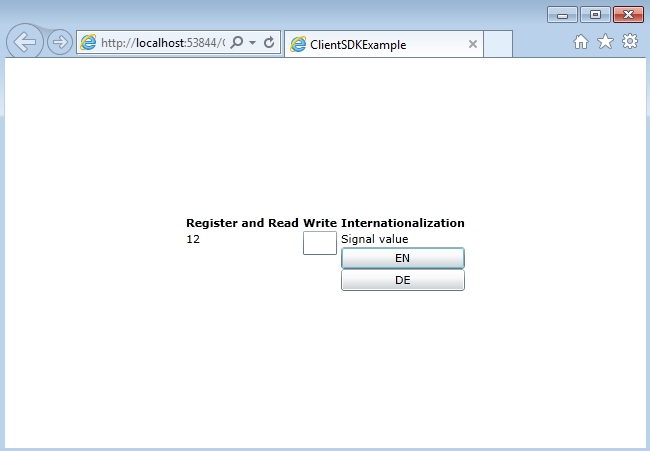
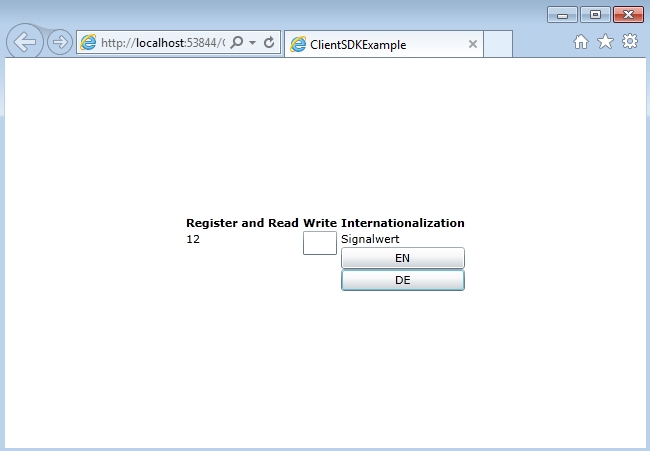
Toggling the languages at run time
The final solution is available for download here:
Make sure to unblock any downloaded file before using it. Windows automatically blocks the files coming from the internet for security reasons.
If downloading a Visual Studio solution, make sure to update all the references marked with the warning symbol before building it.