Write operations
Check out this article and learn more details about the WEBfactory 2010 Client SDK write operations and the involved paramters.
The following article documents the Write methods from the WEBfactory 2010 Silverlight SDK. To view an example on how to use the Write methods, click on the link below:
WriteSignal
Method Definition
void WriteSignal(string serverName, string signalName, object value); void WriteSignal(string serverName, string signalName, object value, OnResultDelegate resultDelegate); void WriteSignal(string serverName, string signalName, object value, string user, string password); void WriteSignal(string serverName, string signalName, object value, string user, string password, bool isDomainUser); void WriteSignal(string serverName, string signalName, object value, string user, string password, OnResultDelegate resultDelegate); void WriteSignal(string serverName, string signalName, object value, string user, string password, bool isDomainUser, OnResultDelegate resultDelegate); void WriteSignal(string serverName, string signalName, object value, string password); void WriteSignal(string serverName, string signalName, object value, string password, OnResultDelegate resultDelegate);
Calling Parameters
serverName Host name of WEBfactory 2010 server
signalName Signal name
value Signal value that should be written
user User name
password User password
isDomainUser User is in domain
resultDelegate Delegate to function handling the result
Description
This method writes a value to a signal.
serverName is necessary for multi server systems only. For a single server project it can be empty.
The delegate OnResultDelegate is defined as:
void OnResultDelegate(Exception error, int[] results);
The error (type Exception) is a Silverlight error indicator for asynchronous operations. WEBfactory 2010 bypasses this exception to SDK users to make it easier to find communication errors.
Each value of the integer array results may have one of the following values:
0: Success
-4095: User login failed (Login)
-4094: No user logged in (Write)
-4093: Insufficient user authorizations (Write)
-4092: User already logged on (Login)
-4091: Invalid user password (Login)
-4090: Max user count reached (Login)
-4089: Unspecified server error (Login, Write)
-4088: Signal is write protected (Write)
ToggleSignal
Method Definition
void ToggleSignal(string serverName, string signalName, object onValue, object offValue); void ToggleSignal(string serverName, string signalName, object onValue, object offValue, OnResultDelegate resultDelegate);
Calling Parameters
serverName Host name of WEBfactory 2010 server
signalName Signal name
onValue Signal value for on state
offValue Signal value for off state
resultDelegate Delegate to function handling the result
Description
This method is used for toggle buttons. It internally uses a WriteSignal operation. If the current value is offValue it writes onValue to the signal otherwise onValue.
serverName is necessary for multi-server systems only. For a single server project it can be empty.
The signal needs to be a registered signal (refer to RegisterSignal or RegisterSignalChangedHandler for details).
There are two methods available. The difference is that one method is able to register a callback which indicates the result of the operation.
The delegate OnResultDelegate is defined as:
void OnResultDelegate(Exception error, int[] results);
The error (type Exception) is a Silverlight error indicator for asynchronous operations. WEBfactory 2010 bypasses this exception to SDK users to make it easier to find communication errors.
Each value of the integer array results may have one of the following values:
0: Success
-4095: User login failed (Login)
-4094: No user logged in (Write)
-4093: Insufficient user authorizations (Write)
-4092: User already logged on (Login)
-4091: Invalid user password (Login)
-4090: Max user count reached (Login)
-4089: Unspecified server error (Login, Write)
-4088: Signal is write protected (Write)
Write operations tutorials
Check out these easy step based guides in order to learn how te WEBfactory 2010 Client SDK write operations work.
In the following tutorial, you will learn to extend the ClientSDKExample Silverlight Application to also write values to signals, using the WEBfactory 2010 Client SDK Write operations. To achieve this, we will use the writable Setpoint 2 signal from the WEBfactory 2010 Demo database, also used in the previous Register and Read operations example tutorial, and a new TextBox control.
The following tutorial is based on the solution build in the Register and Read operations example tutorial.
In order to complete the steps described below, WEBfactory 2010 Server must be running and the Demo database must be loaded in WEBfactory 2010Studio.
All versions of Microsoft Visual Studio and Microsoft Expression Blend can be used to create this example. For extended compatibility, we will use Microsoft Visual Studio 2010.
The guide is split in three sections:
The final solution is available for download here:
Make sure to unblock any downloaded file before using it. Windows automatically blocks the files coming from the internet for security reasons.
If downloading a Visual Studio solution, make sure to update all the references marked with the warning symbol before building it.
Editing the XAML
To demonstrate the Write operations available in the Client SDK, we first need to add a new TextBox control, which will allow us to input values. The same operations need to be performed in both Visual Studio and Expression Blend.
Using Microsoft Visual Studio
In the ClientSDKExample solution, open the MainPage.xaml file.
In the horizontal StackPanel container, add a new vertical StackPanel to hold our new controls. Set the Margin attribute to 2.
<StackPanel Orientation="Vertical" Margin="2"> </StackPanel>
In this StackPanel, add a new TextBlock with the Text attribute Write. Also add a TextBox with the Name attribute SignalInput.
<StackPanel Orientation="Vertical" Margin="2"> <TextBlock Text="Write" FontWeight="Bold"></TextBlock> <TextBox Name="SignalInput"></TextBox> </StackPanel>
Define the KeyUp event for the SignalInput TextBox control and name it SignalInput_KeyUp:
<StackPanel Orientation="Vertical" Margin="2"> <TextBlock Text="Write" FontWeight="Bold"></TextBlock> <TextBox Name="SignalInput" KeyUp="SignalInput_KeyUp"></TextBox> </StackPanel>
Using Microsoft Expression Blend
Drag a new TextBlock control on the MainPage and change its default text to Write. This will act as a label for our demonstrated operation.
Add a new TextBox under the new TextBlock. Using the Name property, name the TextBox as SignalInput.
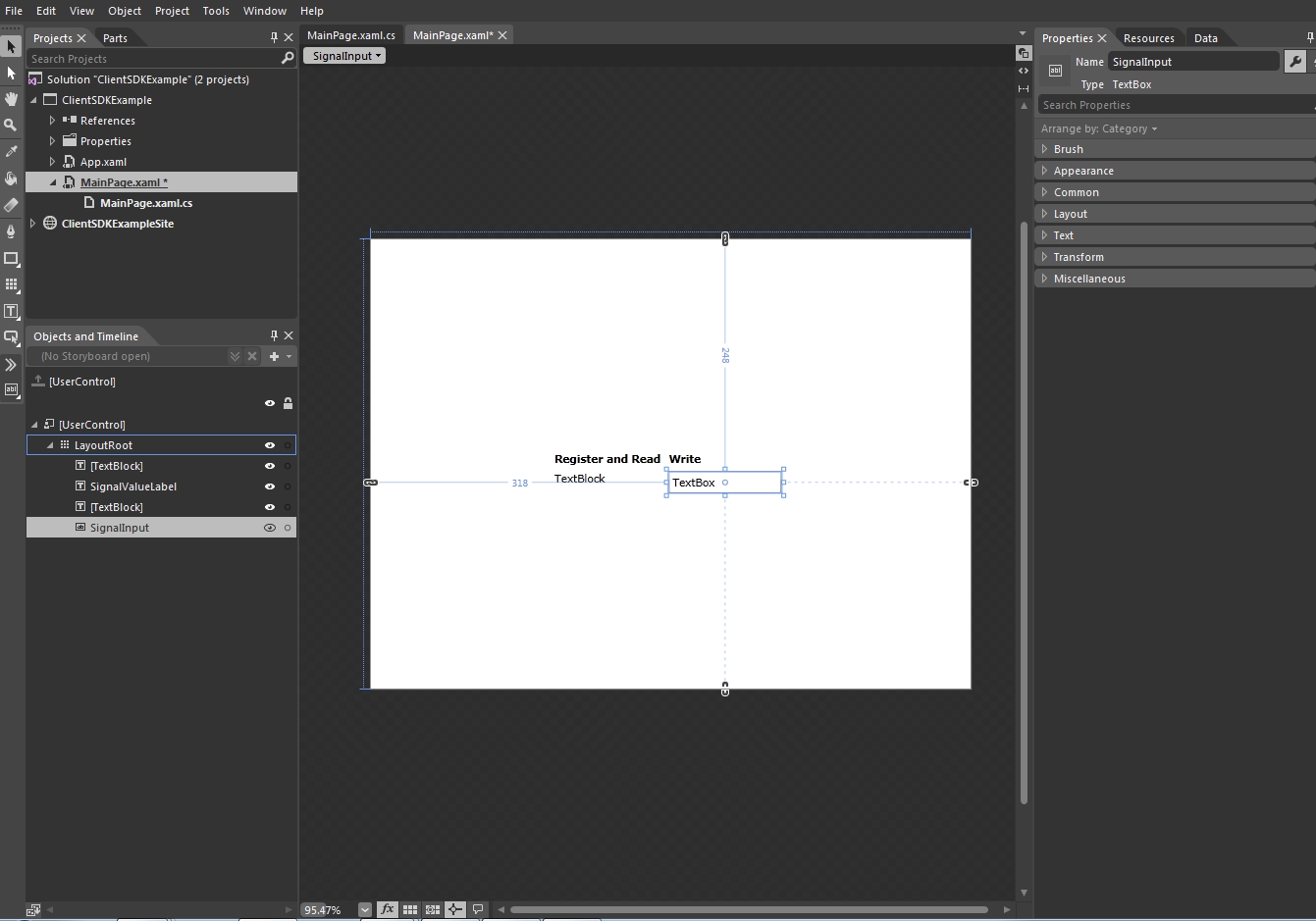
While having the SignalInput TextBox selected, go in the Event handlers tab of the Properties panel and double-click on the KeyUp event. This will create the event handler for the KeyUp event, which we will use later in the code.
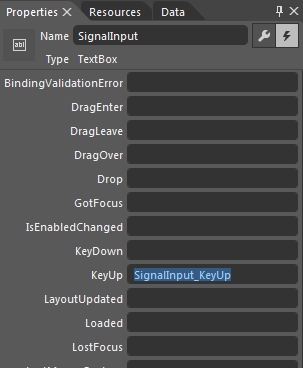
Programming the application
The programming part of the ClientSDKExample goes the same for both Microsoft Visual Studio and Expression Blend, though it is highly recommended to use Microsoft Visual Studio for programming.
Use the Solution Explorer to open the MainPage.xaml.cs.
We need to define the new SignalInput_KeyUp event and write the value from the TextBox to the specified signal when the event occurs.
private void SignalInput_KeyUp(object sender, KeyEventArgs e) { }
Inside the SignalInput_KeyUp event, make a condition so that the action is executed only when the Enter key is used (pressed then released):
private void SignalInput_KeyUp(object sender, KeyEventArgs e) { if (e.Key == Key.Enter) { } }
Now we need to define the action. In our case, we will use the
WriteValue()
method from the connector to write the value from the Text property of the SignalInput TextBox to the signal defined by the SignalName constant and validate the writing of the signal using theWriteSignalHandler()
method:private void SignalInput_KeyUp(object sender, KeyEventArgs e) { if (e.Key == Key.Enter) { wfConnector.WriteSignal("", SignalName, SignalInput.Text, WriteSignalHandler); } }
Next we need to create the
WriteSignalHandler()
validation method. It will display an error message with the relevant error code if the writing was not successful:private void WriteSignalHandler(Exception error, int[] results) { var errorCode = results == null ? 0 : results.FirstOrDefault(); if (!IsWritingSuccessful(error, results)) { string errorMessage = error != null ? error.Message : "Write failed with error code: " + errorCode; MessageBox.Show(errorMessage); } }
As the
WriteSignalHandler()
method uses theIsWritingSuccessful()
method to check if any error code was returned when writing the signal value, we need to define it:private bool IsWritingSuccessful(Exception error, IEnumerable<int> results) { var errorCode = results == null ? 0 : results.FirstOrDefault(); return error == null && errorCode == 0; }
Building and testing the application
Press F5 to build and run the solution. Make sure that the correct database (containing the used signals) is loaded and the WEBfactory 2010 Server is started.
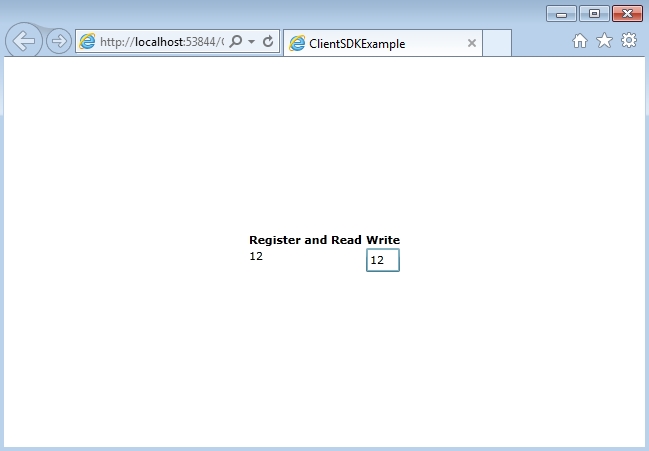
The final solution is available for download here:
Make sure to unblock any downloaded file before using it. Windows automatically blocks the files coming from the internet for security reasons.
If downloading a Visual Studio solution, make sure to update all the references marked with the warning symbol before building it.