Working with SignalInformationChanged
Check out this article and learn more details about working with SignalInformationChanged source codes.
In this example, we will demonstrate how to use the SignalInformationChangeHandler method.
IMPORTANT: The following guide works for WEBfactory 2010 version 3.3. For older versions, the class WFSignalDefinition must be renamed to WFSignalInformation.
Sample files
The example solution can be downloaded using the link below:
Building the example code source
In the following example, we will create a simple control that will display signal information. The displayed signal information will be the signal name. For this example, we will use the Setpoint 1 signal.
Create a Silverlight project in Visual Studio.
Reference the WFCore, WFMath and WFShared dll files from the Silverlight/Standard folder (from the WEBfactory 2010 installation folder).
Create a new class named SignalInformationDisplay and open its code source. Make sure you have the correct usings in the code source:
using System; using System.Linq; using System.Windows.Controls; using WFSilverlight.Core;
Declare the SignalName constant and the connector (here we state the signal Setpoint 1):
private const string SignalName = "Setpoint 1"; private IWFConnector connector;
Define the constructor:
public SignalInformationDisplay() { InitializeComponent(); InitializeConnector(); }
Now it's time to build the event handler. For the event handler, there are two possibilities:
IMPORTANT: For WEBfactory 2010 versions older than 3.3, use WFSignalInformation instead of WFSignalDefinition.
The first option (the recommended option) is to build a complete event handler, that will handle a list of signals and signal information instead of just one signal and one signal information. This method allows us to further adapt the code for more signals. In this case, the event handler will search the list of signals received and display the required property for the desired signals.
private void OnSignalInformationChangedHandler(string servername, string[] signalnames, WFSignalDefinition[] signaldefinitions) { for (int i = 0; i < signalnames.Length; i++) { var signalName = signalnames[i]; var signalInformation = signaldefinitions[i]; if (signalName == SignalName) //find the signalDefinition for the correct signalName. { DisplaySignalInformation(signalInformation); break; } } }
The second option is an event handler that takes the first item from the list (in our case Setpoint 1) and displays the required property. This is option is not recommended due to the limitations of using only one existing signal.
private void OnSignalInformationChangedHandler(string servername, string[] signalnames, WFSignalDefinition[] signaldefinitions) { var signalInformation = signaldefinitions.First(); DisplaySignalInformation(signalInformation);
}
Now let's handle the helper methods. We are going to build the InitializeConnector() method, the DisposeConnector() method and the DisplaySignalInformation() method:
private void InitializeConnector() //initializes the connector { connector = new WFConnector(false); connector.RegisterSignalInformationChangedHandler(null, SignalName, OnSignalInformationChangedHandler); //server is null if the targeted webservice is on localhost. } private void DisposeConnector() //the important dispose method { if (connector == null) return; connector.UnregisterSignalInformationChangedHandler(null, SignalName, OnSignalInformationChangedHandler); connector.Dispose(); } private void DisplaySignalInformation(WFSignalDefinition signalInformation) //the method that displays the signal information\ { uxSignalInformation.Text = signalInformation != null //if a signal with that signal name is not found on the server, then a null signalInformation is retrieved ? signalInformation.Name : "Signal information not available for signal " + SignalName; }
The last step has a high importance - implementing the IDisposable. This will keep the memory free of unneeded resources:
/// Flag used to indicate whether the object is disposed or not /// (to prevent double-disposal) private bool isDisposed; /// Disposes of the managed resources. This method /// is not called by the destructor, so it is safe to /// reference other objects. /// Override this method to provide descendent managed resources /// cleanup. protected virtual void DisposeManagedResources() { DisposeConnector(); } /// Disposes of the resources used by this object /// <param name="disposing"> /// <b>true</b> if the dispose is not called explicitly /// due to IDisposable implementation; <b>false</b> otherwise. /// If this flag is set to true, it is safe to reference other /// objects; otherwise it isn't. /// </param> protected virtual void Dispose(bool disposing) { if (!isDisposed) // only dispose once! { if (disposing) { DisposeManagedResources(); } } isDisposed = true; } /// Implementation of IDisposable.Dispose. It frees any resources /// related to this object and prevents the garbage collector to /// finalize the object. public void Dispose() { Dispose(true); // tell the GC not to finalize GC.SuppressFinalize(this); }
Building the XAML
In the SignalInformationDisplay XAML file, we will place a simple text block that will display our signal name:
<UserControl x:Class="SignalInfo.SignalInformationDisplay" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"> <Grid VerticalAlignment="Center" HorizontalAlignment="Center"> <TextBlock x:Name="uxSignalInformation"/> <!--here we will display the signal name--> </Grid> </UserControl>
The final output
When running the project, the output window will display the signal name, centered in the middle of the page:
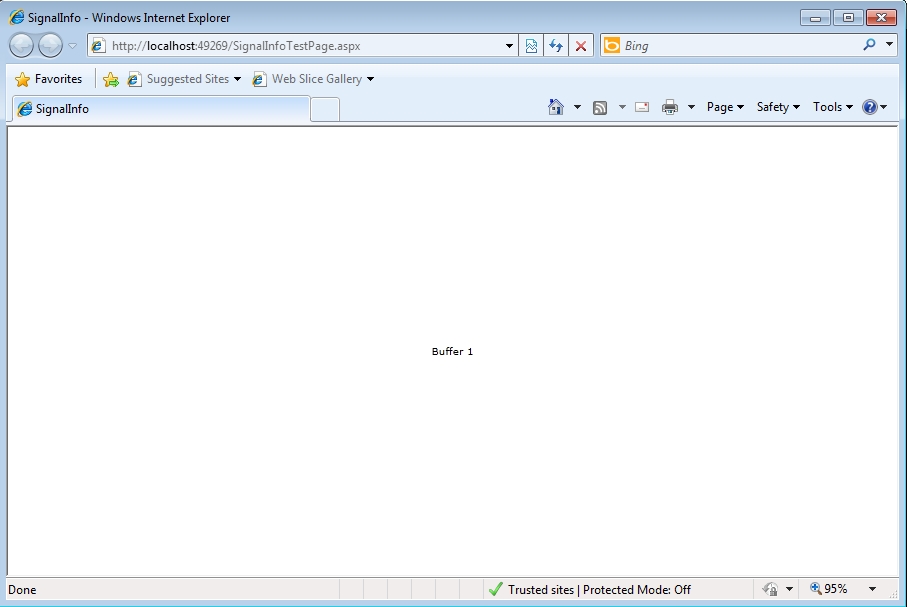
Displaying the signal name